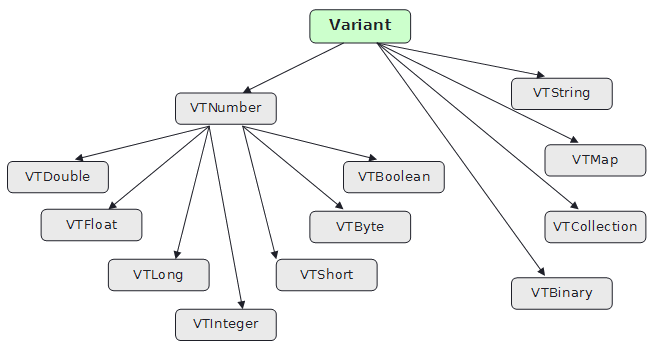
Why is this useful?
- It separates the data structure from the representation so you are not locked into one representation (e.g. XML).
- It is an extremely easy way to serialize data structures, from a simple property map to a complex object tree.
- It provides different serializations for different uses:
- Binary for efficient storage,
- Java/C-style syntax for a compact representation that is human readable,
- XML for compatibility.
- It provides a validation/schema mechanism defined as code (ie. it separates the schema definition from the format).
- It provides an object->variant->object mapper for simple serialization.
package org.boris.variantcodec; import org.boris.variant.VTCollection; import org.boris.variant.VTMap; import org.boris.variant.codec.SourceCodec; public class VariantCodecExample { public static void main(String[] args) throws Exception { VTMap m = new VTMap(); m.add("var1", "Hello World!"); m.add("num1", 12); m.add("float1", 23.5f); m.add("binary1", new byte[] { 3, 4, 4, 5, 5 }); VTCollection c = new VTCollection(); c.add(1); c.add(2); c.add(3); m.add("coll1", c); System.out.println(SourceCodec.encodeFormatted(m)); } }This would output the following:
{ var1="Hello World!"; num1=12; float1=23.5f; binary1=<0304040505>; coll1=[1,2,3]; }If the above output was stored in a String, you would decode it using the following code:
VTMap m = (VTMap) SourceCodec.decode(s); System.out.println(m.getString("var1"));This would output the following:
Hello World!